Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
Let’s say you have a Magento multi-website/store installation and you want to assign the products of a particular category to the new store. I wrote a few lines of code to accomplish this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | <?php use MagentoFrameworkAppBootstrap; require __DIR__ . '/../app/bootstrap.php'; $bootstrap = Bootstrap::create(BP, $_SERVER); $objectManager = $bootstrap->getObjectManager(); $state = $objectManager->get('MagentoFrameworkAppState'); $state->setAreaCode('frontend'); $categoryIds = [41]; // Category IDs whose products you want to assign foreach ($categoryIds as $categoryId) { $category = $objectManager->create('MagentoCatalogModelCategory')->load($categoryId); $productCollection = $category->getProductCollection(); foreach ($productCollection as $product) { $productId = $product->getId(); $websiteIds = $product->getWebsiteIds(); $storeIds = $product->getStoreIds(); if (!in_array(1, $websiteIds)) { continue; } $websiteIds[] = 2; $websiteIds = array_unique($websiteIds); if (in_array(2, $storeIds)) { continue; } $storeIds[] = 2; $storeIds = array_unique($storeIds); $product->setWebsiteIds($websiteIds); $product->save(); // Copy the details of the products to the new store $newProduct = $objectManager->create('MagentoCatalogModelProduct')->load($productId) ->setStoreIds($storeIds) ->save(); echo "Done : #$productId"; echo '<br>'; } } |
You can use this piece of code wherever you want in Magento 2. For example, you can use it as a standalone script to update a bunch of products in the /pub folder. Or, you can use it as a product save observer. It’s your call!
This may not be the most optimized solution, but it did the trick for me.
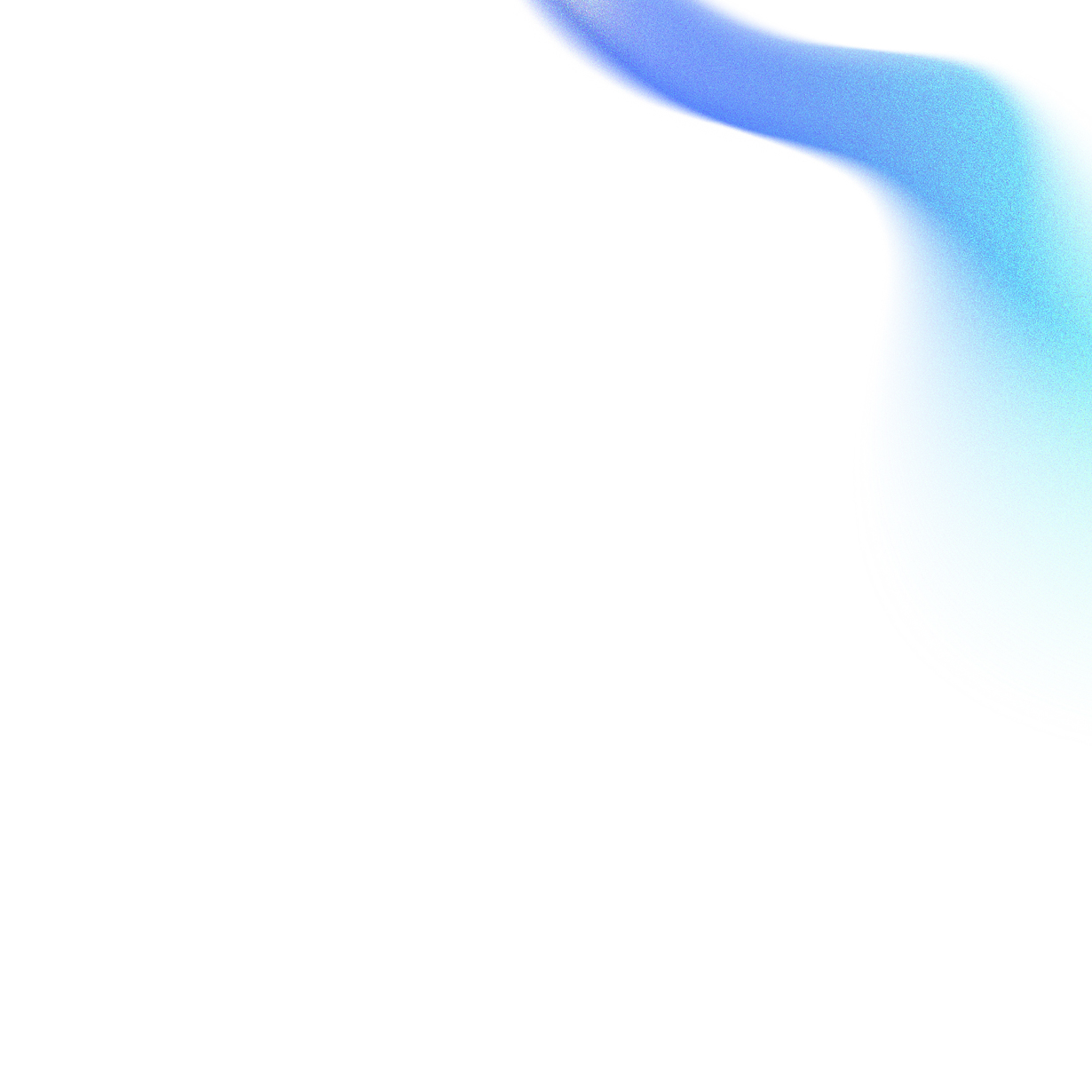
Related Articles
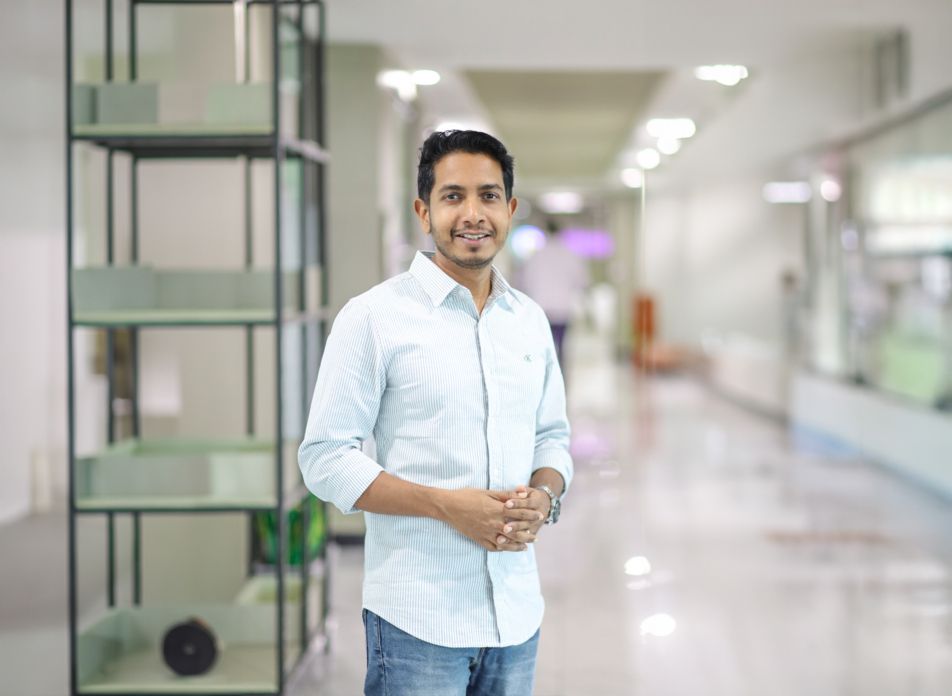