How to Secure and Test Jira Webhooks in Laravel?
Integrating Jira webhooks into your Laravel application can greatly enhance automated workflows. However, developers often face challenges in ensuring the security and reliability of these webhooks. This article highlights common issues faced, along with effective solutions to secure and test Jira webhooks in Laravel.
Issues Faced: Ensuring Security and Reliable Testing
- Unverified Webhook Requests
Many applications process webhook requests without verifying authenticity, leaving the system vulnerable to spoofing or malicious activities.
- Testing Webhooks Locally
Testing Jira webhooks in a local Laravel environment can be challenging due to the lack of direct access to external services.
Solutions: Securing and Testing Jira Webhooks in Laravel
Step 1: Setting Up a Jira Webhook
Before securing and testing the webhooks, we need to set one up in Jira. Follow these steps:
1. Go to Jira’s Webhook Management:
– Navigate to Jira Settings > System > Webhooks.
2. Create a New Webhook:
– Click the Create a Webhook button.
– Provide a descriptive name for the webhook to identify it easily. Eg – Laravel Task Update Webhook.
– Define the URL of your Laravel application where the webhook should be sent.Provide the fully qualified URL (e.g., https://example.com/jira/webhook) of your Laravel application endpoint where the webhook data should be sent. Ensure it is accessible and uses HTTPS for security.
– Select the Jira events (e.g., issue creation, update, etc.) that should trigger the webhook.
3. Webhook URL Security:
– Add a verification token or secret key as a query parameter or in the request header (recommended), and validate it in your Laravel application to confirm the request is genuinely from Jira.
Step 2: Setting Up the Webhook Endpoint in Laravel
Laravel applications need an endpoint to receive the Jira webhook events. Here’s how you can create one:
1. Define the Route:
In routes/web.php, create a route for the webhook.
use App\Http\Controllers\JiraWebhookController;
Route::post(‘/jira-webhook’, [JiraWebhookController::class, ‘handleWebhook’])->name(‘jira.webhook’);
Note : To allow Jira to send requests without requiring a CSRF token, exclude the /jira-webhook route from CSRF verification in the VerifyCsrfToken middleware. This can be done by adding the route to the exception list in the middleware file located at app/Http/Middleware/VerifyCsrfToken.php.
2. Create the Controller:
In app/Http/Controllers, create a JiraWebhookController:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\Log; use Illuminate\Http\JsonResponse; class JiraWebhookController extends Controller { public function handleWebhook(Request $request): JsonResponse { // Log the incoming request Log::info('Received Jira Webhook', ['data' => $request->all()]); // Handle the webhook event // (For now, let's just return a success message) return response()->json(['message' => 'Webhook received successfully!'], 200); } } |
Step 3: Securing the Jira Webhook
Now, we need to ensure the webhook is secure. Jira allows you to secure webhooks using shared secrets, so only authorized requests from Jira can interact with your Laravel application.
1. Generate a Shared Secret:
Create a shared secret that Jira will use to sign the requests
php artisan tinker
Str::random(32);
OR
Alternatively, a secret token can be generated from Jira webhook settings.
Save this secret in your .env file:
JIRA_WEBHOOK_SECRET=your_random_secret
2. Add Signature Verification in Laravel:
In your JiraWebhookController, you’ll verify the incoming request’s signature.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\Log; use Illuminate\Http\JsonResponse; class JiraWebhookController extends Controller { public function handleWebhook(Request $request): JsonResponse { // Validate signature $expectedSignature = 'sha256=' . hash_hmac('sha256', $request->getContent(), env('JIRA_WEBHOOK_SECRET')); $signature = $request->header('X-Hub-Signature-256'); if (!hash_equals($expectedSignature, $signature)) { Log::warning('Invalid Jira webhook signature.'); return response()->json(['message' => 'Unauthorized'], 401); } // Log the valid incoming request Log::info('Received Jira Webhook', ['data' => $request->all()]); // Handle the webhook event return response()->json(['message' => 'Webhook received successfully!'], 200); } } |
Step 4: Testing the Jira Webhook Locally
To test webhooks locally, you can use tools like ngrok to expose your local Laravel application to the internet.
1. Install ngrok:
You can download and install ngrok from its official website.
2. Expose Your Local Laravel App:
Run the following command to expose your local server.
ngrok http 8000
3. Update Jira Webhook URL:
Update the webhook URL in Jira with the new ngrok URL:
https://abcd1234.ngrok.io/jira-webhook
Step 5: Handling Different Jira Events
In the handleWebhook method, you can inspect the payload and add logic to handle specific events.
For example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | public function handleWebhook(Request $request): JsonResponse { $eventType = $request->header('X-Event-Key'); // Jira sends the event type in the headers switch ($eventType) { case 'jira:issue_created': // Handle issue creation Log::info('Issue created', ['data' => $request->all()]); break; case 'jira:issue_updated': // Handle issue updates Log::info('Issue updated', ['data' => $request->all()]); break; // Add more cases as needed } return response()->json(['message' => 'Webhook processed!'], 200); } |
Step 6: Testing Webhook Security
To test the webhook’s security, you can try sending a request to the webhook endpoint from Postman or another HTTP client without including the signature header. Your Laravel application should reject the request with a 401 Unauthorized error. Ensure you use HTTPS for secure testing, especially in production environments.”
You can also use Postman’s Pre-request Script feature to simulate the signing process and test the webhook endpoint’s signature verification.
By addressing these issues with the outlined solutions, you can effectively secure and test Jira webhooks in Laravel, ensuring robust and reliable integration for your workflows. Contact a Laravel Development Agency.
Recent help desk articles
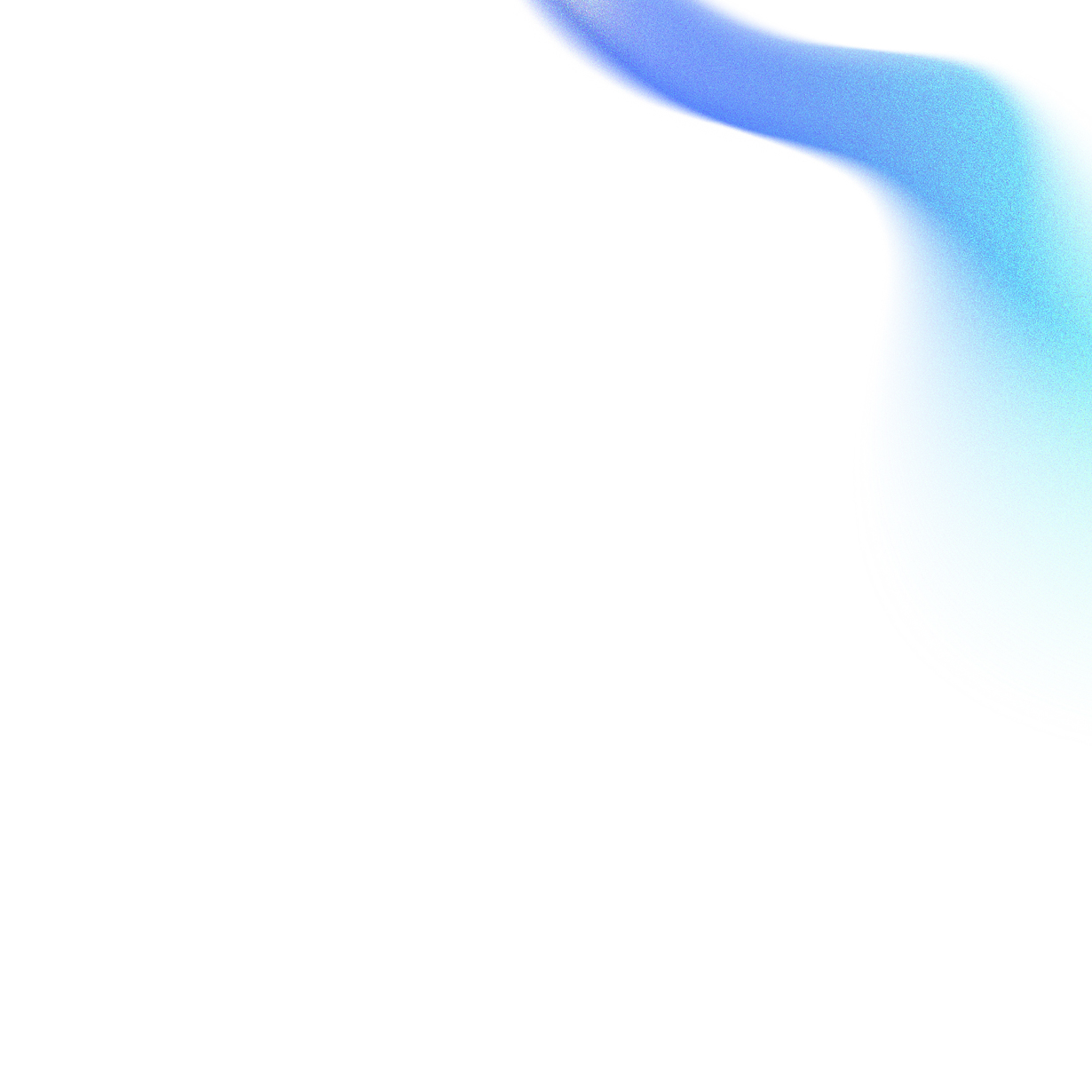
Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
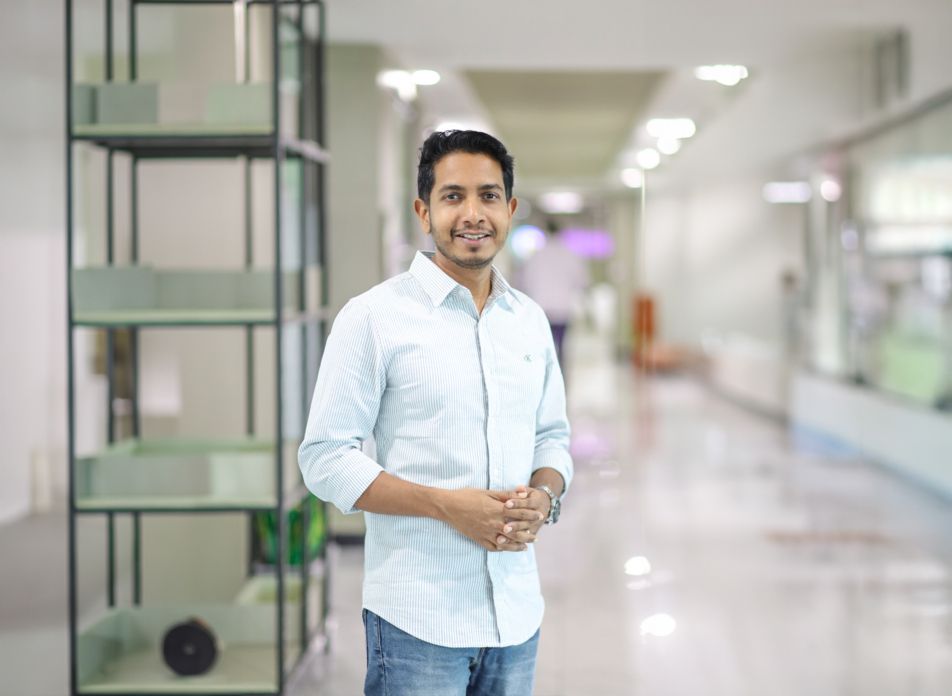