Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
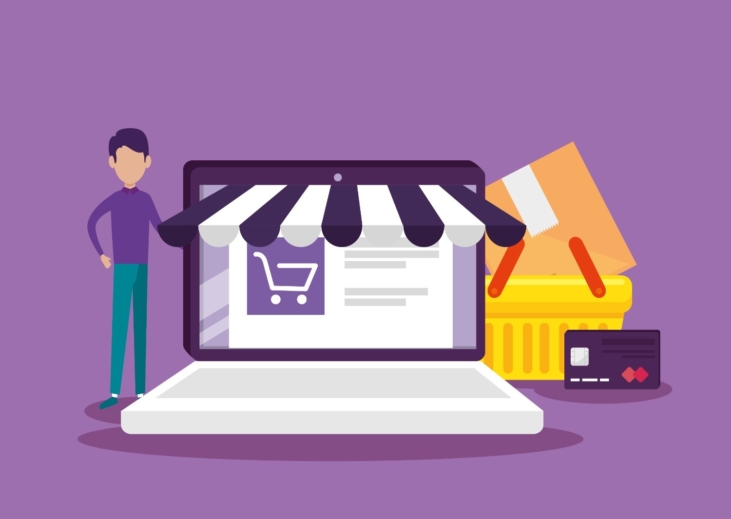
Ever found yourself feeling constrained by traditional e-commerce platforms?
You’re not alone. As brands push for more unique digital experiences, the limitations of conventional storefronts become increasingly apparent. That’s where headless commerce architecture comes in, specifically BigCommerce’s Storefront API.
As someone who’s implemented dozens of headless BigCommerce solutions, we’ve seen firsthand how this approach can transform businesses.
Let’s dive into how you can harness this powerful API to create exceptional customer experiences.
What is BigCommerce Storefront API?
The BigCommerce Storefront API is a GraphQL-based API that allows developers to access store data for creating custom shopping experiences. Unlike the older REST APIs, this GraphQL implementation gives you precise control over what data you retrieve, making your applications faster and more efficient.
Think of it as having direct access to your store’s engine, allowing you to build any interface you want on top of BigCommerce’s robust commerce platform.
Key Features:
- GraphQL Implementation: Request exactly the data you need, nothing more
- Real-time Data: Access up-to-date product, customer, and order information
- Customization: Build unique frontend experiences with any technology
- Performance: Optimize API calls for faster loading times
Pro-tip: When starting with BigCommerce’s Storefront API, invest time in learning GraphQL fundamentals. This will dramatically improve your query efficiency and application performance.
Why Go Headless with BigCommerce?
Before implementing, let’s understand why you might choose a headless approach with BigCommerce.
Traditional e-commerce platforms bundle the front-end (what customers see) with the back-end (the commerce engine). Headless architecture separates these, giving you:
- Creative Freedom: Build your storefront with any front-end technology (React, Vue, Next.js, etc.)
- Omnichannel Capabilities: Deploy to web, mobile apps, kiosks, voice assistants, and more from a single back-end
- Performance Optimization: Create lightning-fast experiences with modern front-end frameworks
- Future-Proofing: Adopt new technologies without rebuilding your entire commerce platform
Is your e-commerce platform holding you back?
Setting Up Your Headless BigCommerce Environment
Let’s get practical. Here’s how to set up your development environment for working with the BigCommerce Storefront API.
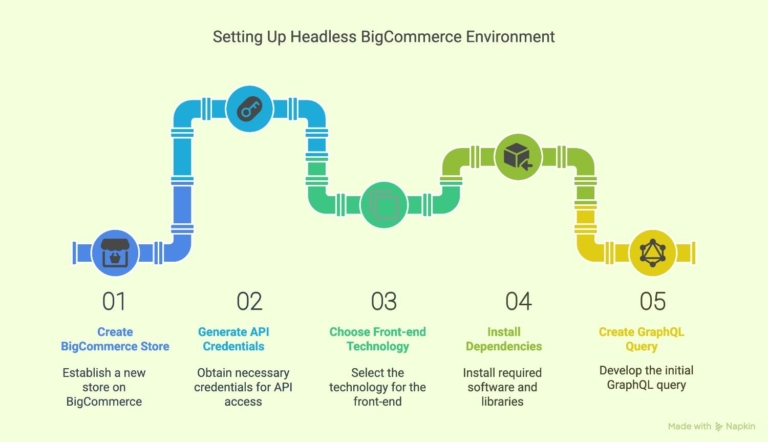
Step 1: Create a BigCommerce Store and Generate API Credentials
- Sign up for a BigCommerce account
- Navigate to Advanced Settings > API Accounts
- Create a Storefront API Token with appropriate scopes
1 2 3 4 | // Example of storing your credentials (in a secure environment variable) const STOREFRONT_API_TOKEN = process.env.STOREFRONT_API_TOKEN; const STORE_HASH = process.env.STORE_HASH; |
Step 2: Choose Your Front-end Technology
While you can use any frontend technology, these are particularly well-suited for headless commerce:
Framework | Pros | Cons | Ideal For |
---|---|---|---|
Next.js | SEO-friendly, SSR support | Steeper learning curve | Content-heavy stores |
React | Component reusability, large ecosystem | Requires additional SEO work | Custom interfaces |
Vue.js | Easy to learn, lightweight | Smaller ecosystem than React | Smaller projects |
Gatsby | Performance, static generation | Build times can be long | Catalog-focused sites |
At 2HatsLogic, we’ve found Next.js to be the most versatile choice for most headless BigCommerce projects due to its hybrid rendering capabilities.
Step 3: Install Required Dependencies
1 2 3 4 | # For a Next.js project npm init npm install next react react-dom graphql graphql-request |
Step 4: Create Your First GraphQL Query
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | import { GraphQLClient } from 'graphql-request'; // Create a GraphQL client const client = new GraphQLClient( `https://store-${STORE_HASH}.mybigcommerce.com/graphql`, { headers: { 'Authorization': `Bearer ${STOREFRONT_API_TOKEN}` }, } ); // Example query to fetch products const PRODUCTS_QUERY = ` query { site { products (first: 10) { edges { node { name sku path prices { price { value currencyCode } } } } } } } `; // Function to fetch products async function fetchProducts() { const data = await client.request(PRODUCTS_QUERY); return data.site.products.edges; } |
Need expert guidance on implementing headless commerce?
Building Core E-commerce Functionality
Once your environment is set up, you’ll need to implement essential e-commerce functionality using the Storefront API.
Product Catalog Implementation
The heart of any e-commerce site is its product catalog. Here’s how to build a robust catalog with the Storefront API:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | // Query for a product catalog with filtering and pagination const CATALOG_QUERY = ` query CatalogProducts($categoryId: Int, $first: Int!, $after: String) { site { category(entityId: $categoryId) { name products(first: $first, after: $after) { pageInfo { hasNextPage endCursor } edges { node { entityId name path defaultImage { url(width: 300) altText } prices { price { value currencyCode } salePrice { value currencyCode } } } } } } } } `; |
For better performance, consider:
- Implementing pagination to load products as users need them
- Using image optimization with the width parameter
- Caching frequent queries with a solution like Redis
Shopping Cart Functionality
The cart is where the Storefront API shines compared to traditional implementations:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | // Add to cart mutation const ADD_TO_CART = ` mutation AddToCart($productId: Int!, $variantId: Int!, $quantity: Int!) { cart { addCartLineItems( input: { cartLineItems: [ { quantity: $quantity productEntityId: $productId variantEntityId: $variantId } ] } ) { cart { id lineItems { physicalItems { name quantity } } } } } } `; |
Line breaks make content easier to digest, especially technical content.
Let’s add another useful feature:
Implementing Real-time Inventory Updates
One advantage of headless architecture is the ability to display real-time inventory information:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | const INVENTORY_QUERY = ` query ProductInventory($productId: Int!) { site { product(entityId: $productId) { inventory { isInStock aggregated { availableToSell } } variants { edges { node { entityId inventory { isInStock aggregated { availableToSell } } } } } } } } `; |
Pro tip: For high-traffic sites, implement a caching layer for inventory data with a short TTL (Time To Live) of 1-2 minutes. This provides near-real-time inventory while reducing API load.
Transform your e-commerce performance with a headless approach.
Advanced Implementation Strategies
Once you’ve mastered the basics, these advanced techniques will elevate your headless implementation:
1. Custom Checkout Experience
While BigCommerce provides an embedded checkout option, building a custom checkout with the Storefront API gives you complete control:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | // Initialize checkout const CHECKOUT_MUTATION = ` mutation CreateCheckout($cartId: String!) { checkout { createCheckout(input: { cartId: $cartId }) { checkout { id webUrl } checkoutMutationError { code message } } } } `; |
Remember that handling payment security is critical. Consider using BigCommerce’s hosted payment components even within your custom checkout.
2. Content + Commerce Integration
A major benefit of headless architecture is the ability to integrate content seamlessly:
Common content integration patterns include:
- Content-enriched product pages: Combine product data from BigCommerce with marketing content from your CMS
- Shoppable blog posts: Add shopping functionality directly to content pages
- Unified search: Search across products and content simultaneously
3. Performance Optimization Techniques
Headless implementations can be blazing fast with these optimizations:
- Implement ISR (Incremental Static Regeneration) with Next.js for product pages
- Use edge caching for API responses
- Optimize images using modern formats and lazy loading
- Employ GraphQL fragments to reuse common query parts
Ready to optimize your e-commerce performance?
Common Challenges and Solutions
Implementing a headless architecture with BigCommerce Storefront API isn’t without challenges. Here are solutions to the most common issues:
Challenge 1: Managing Cart State Across Devices
Solution: Implement a hybrid cart approach:
1 2 3 4 5 6 7 8 9 10 | // Function to merge anonymous cart with user cart after login async function mergeCartsAfterLogin(anonymousCartId, userToken) { const mergeResult = await client.request(MERGE_CARTS_MUTATION, { anonymousCartId, userToken }); return mergeResult.cart.id; } |
Challenge 2: SEO Management
Solution: Implement server-side rendering for critical pages and utilize structured data:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | // Product structured data example function generateProductJsonLd(product) { return { '@context': 'https://schema.org/', '@type': 'Product', name: product.name, image: product.images.map(img => img.url), description: product.description, sku: product.sku, offers: { '@type': 'Offer', url: `https://yourdomain.com/products/${product.slug}`, priceCurrency: product.price.currencyCode, price: product.price.value, availability: product.inventory.isInStock ? 'https://schema.org/InStock' : 'https://schema.org/OutOfStock' } }; } |
Challenge 3: Managing Complex Product Options
Solution: Build a dedicated product options selector component:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | // React component for handling product options (simplified) function ProductOptionsSelector({ product, onVariantSelect }) { const [selectedOptions, setSelectedOptions] = useState({}); useEffect(() => { // Find matching variant based on selected options const matchedVariant = findMatchingVariant(product, selectedOptions); if (matchedVariant) { onVariantSelect(matchedVariant); } }, [selectedOptions]); return ( <div className="product-options"> {product.options.map(option => ( <div key={option.id} className="option-group"> <h4>{option.displayName}</h4> <div className="option-values"> {option.values.map(value => ( <button key={value.id} className={selectedOptions[option.id] === value.id ? 'selected' : ''} onClick={() => setSelectedOptions({ ...selectedOptions, [option.id]: value.id })} > {value.label} </button> ))} </div> </div> ))} </div> ); } |
Future-Proofing Your Headless Architecture
The e-commerce landscape continues to evolve rapidly. Here’s how to ensure your headless implementation remains cutting-edge:
1. Embrace Composable Commerce
Beyond headless, consider moving toward a fully composable approach where you select best-of-breed solutions for each commerce function.
2. Implement Progressive Web App (PWA) Capabilities
Enhance your headless storefront with PWA features:
1 2 3 4 5 6 7 8 9 10 11 | // next.config.js example for PWA configuration const withPWA = require('next-pwa'); module.exports = withPWA({ pwa: { dest: 'public', register: true, skipWaiting: true, } }); |
3. Prepare for Web3 and Emerging Technologies
The Storefront API architecture makes it easier to integrate with emerging technologies:
- NFT ownership verification for exclusive products
- AR product visualization using mobile devices
- Voice commerce integration
Want to stay ahead of e-commerce trends?
Conclusion
Implementing BigCommerce’s Storefront API in a headless architecture opens up incredible possibilities for creating unique, high-performance shopping experiences.
By separating your frontend from the commerce engine, you gain the flexibility to innovate while leveraging BigCommerce’s robust commerce capabilities.
At 2HatsLogic, we’ve helped numerous businesses transform their digital commerce with headless architecture.
Our expertise in BigCommerce’s Storefront API, combined with modern frontend technologies, allows us to create exceptional shopping experiences. Contact 2HatsLogic today to discuss your headless commerce project!
FAQ
What is the difference between BigCommerce's Storefront API and its REST API?
The Storefront API uses GraphQL, allowing you to request exactly the data you need in a single call. The REST API requires multiple endpoints for complex operations and returns fixed data structures. For headless implementations, the Storefront API typically offers better performance and flexibility.
Can I use BigCommerce's Storefront API with any front-end framework?
Yes, the Storefront API is framework-agnostic. While popular choices include React, Vue, and Angular, you can use any JavaScript framework or even native mobile development platforms.
How does the Storefront API handle product variants?
The Storefront API provides robust support for variants through its product options structure. You can query available options, their values, and corresponding variant data, including inventory and pricing.
Is it possible to use BigCommerce's checkout with a custom storefront?
Yes, BigCommerce offers an embedded checkout option that you can use with your headless storefront. Alternatively, you can build a completely custom checkout experience using the Storefront API.
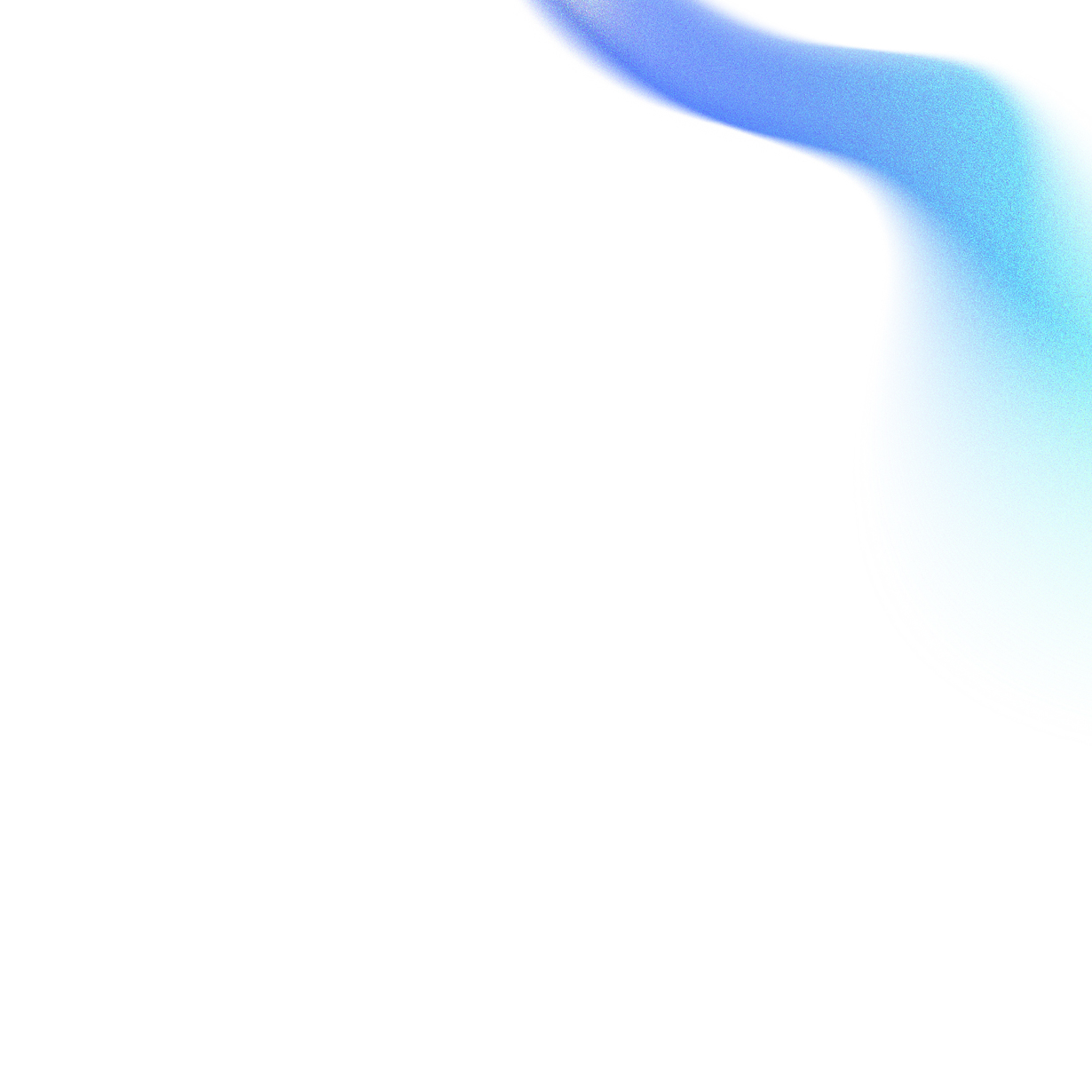
Related Articles
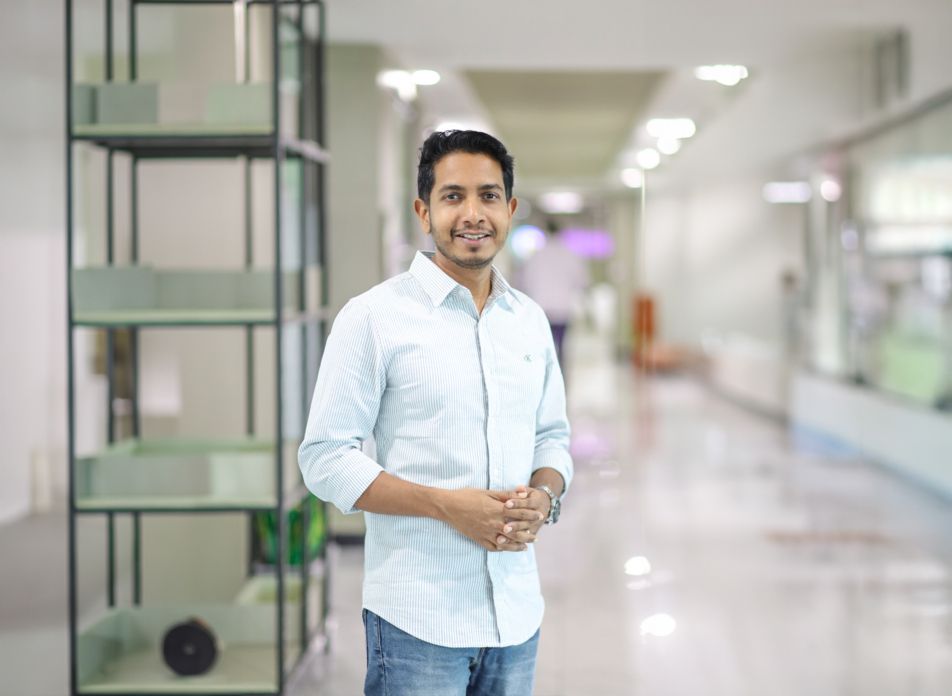