How to Create Filters in Laravel?
Filters are the basics in Laravel that are used for query refinement and efficient data handling. Relationships, query builders, and even pagination experience difficulties during development. This article elaborates on common problems and their solutions while creating filters in Laravel.
Common Issues When Creating Filters in Laravel
1. Incorrect Relationships
Defining relationships with incorrect keys can lead to issues where the queries fail or get the wrong results. For instance:
Using ‘bath_id‘ instead of ‘id‘ in the ProductCategory and ProductType models will cause broken relations.
1 2 3 4 5 | // Incorrect relationship in ProductCategory model public function products() { return $this->hasMany(Product::class, 'bath_id'); // 'bath_id' does not exist } |
2. Typos in Foreign Key Names
A simple typo: the model ProductImages now has a column for ‘propuct_id‘ instead of ‘product_id‘, disrupts the model relationships.
1 2 3 4 | // In the ProductImages model public function product() { return $this->belongsTo(Product::class, 'propuct_id'); // Typo in 'product_id' } |
3. Misuse of Query Builder in Filters
In a custom filter class, using $this->query instead of $this->builder prevents query modification.
1 2 3 | public function title($name) { return $this->query->where('name', 'like', $name . '%'); // Wrong property: should be $this->builder } |
4. Incorrect Pagination Syntax
Passing unnecessary arguments like ‘page‘ to the paginate method results in unexpected pagination behavior.
Solutions to Filter Issues
1. Fix Relationships
Ensure foreign keys are correctly defined:
1 2 3 4 5 6 7 8 9 10 | // In ProductCategory model public function products() { return $this->hasMany(Product::class, 'category_id', 'id'); // 'category_id' is the foreign key in the 'products' table, and 'id' is the primary key in this model. } // In ProductImages model public function product() { return $this->belongsTo(Product::class, 'product_id'); // Fix the typo in foreign key } |
2. Correct Filter Syntax
Replace $this->query with $this->builder in filter methods:
// Custom filter class (e.g., ProductFilter.php)
public function title($name) {
return $this->builder->where(‘name’, ‘like’, $name . ‘%’);
}
3. Use Proper Pagination
Eliminate redundant parameters in the paginate method:
1 2 3 4 5 | // Fetch products with filters and pagination $products = Product::filter($filter) ->with(['category', 'type', 'images']) // Eager loading relationships ->orderBy('name', 'asc') // Sort by product name ->paginate(40); // Limit to 40 results per page |
4. Validate Relationships
Test relationships and filter queries independently to identify errors. Use Laravel Tinker or PHPUnit for debugging.
Example: Debugging with Laravel Tinker
1 2 | php artisan tinker >>> Product::with('category')->filter($filter)->toSql(); |
Example: Testing with PHPUnit
1 2 3 4 5 6 | public function testProductFilterByCategory() { $filter = ['category' => 'Electronics']; $products = Product::filter($filter)->get(); $this->assertCount(10, $products); // Validate the expected number of results } |
Pro Tips for Better Filters
- Stick to Conventions
Avoid making mistakes by following Laravel’s field and relation naming conventions.
- Utilize Query Scopes
Wrap the repetitive query logic within the model scopes.
1 2 3 4 5 6 7 | // In Product model public function scopeFilterByCategory($query, $categoryId) { return $query->where('category_id', $categoryId); } // Usage $products = Product::filterByCategory(1)->get(); |
- Implement Chainable Filter Classes
Use custom filter classes to organize and manage filter logic.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | // app/Filters/ProductFilter.php namespace App\Filters; use Illuminate\Database\Eloquent\Builder; class ProductFilter { protected $builder; public function apply(Builder $builder, $filters) { $this->builder = $builder; foreach ($filters as $filter => $value) { if (method_exists($this, $filter)) { $this->$filter($value); } } return $this->builder; } public function category($categoryId) { return $this->builder->where('category_id', $categoryId); } public function priceRange($min, $max) { return $this->builder->whereBetween('price', [$min, $max]); } } |
Code Example: Applying the Filter
1 2 3 4 5 6 7 | // In Controller $filters = [ 'category' => 1, 'priceRange' => [100, 500] ]; $products = Product::filter(new ProductFilter, $filters)->get(); |
- Debug with Laravel Debugbar
To identify problems with queries and performance, use Debugbar.
- Automate Tests:
Automate the tests of your filters to ensure consistency.
Conclusion
Creating filters in Laravel is straightforward when best practices are followed, but challenges like incorrect relationships or typos can complicate the process. The solutions and tips shared can help you implement effective filters in your Laravel projects.
For advanced assistance, consider hiring Laravel developers.
Recent help desk articles
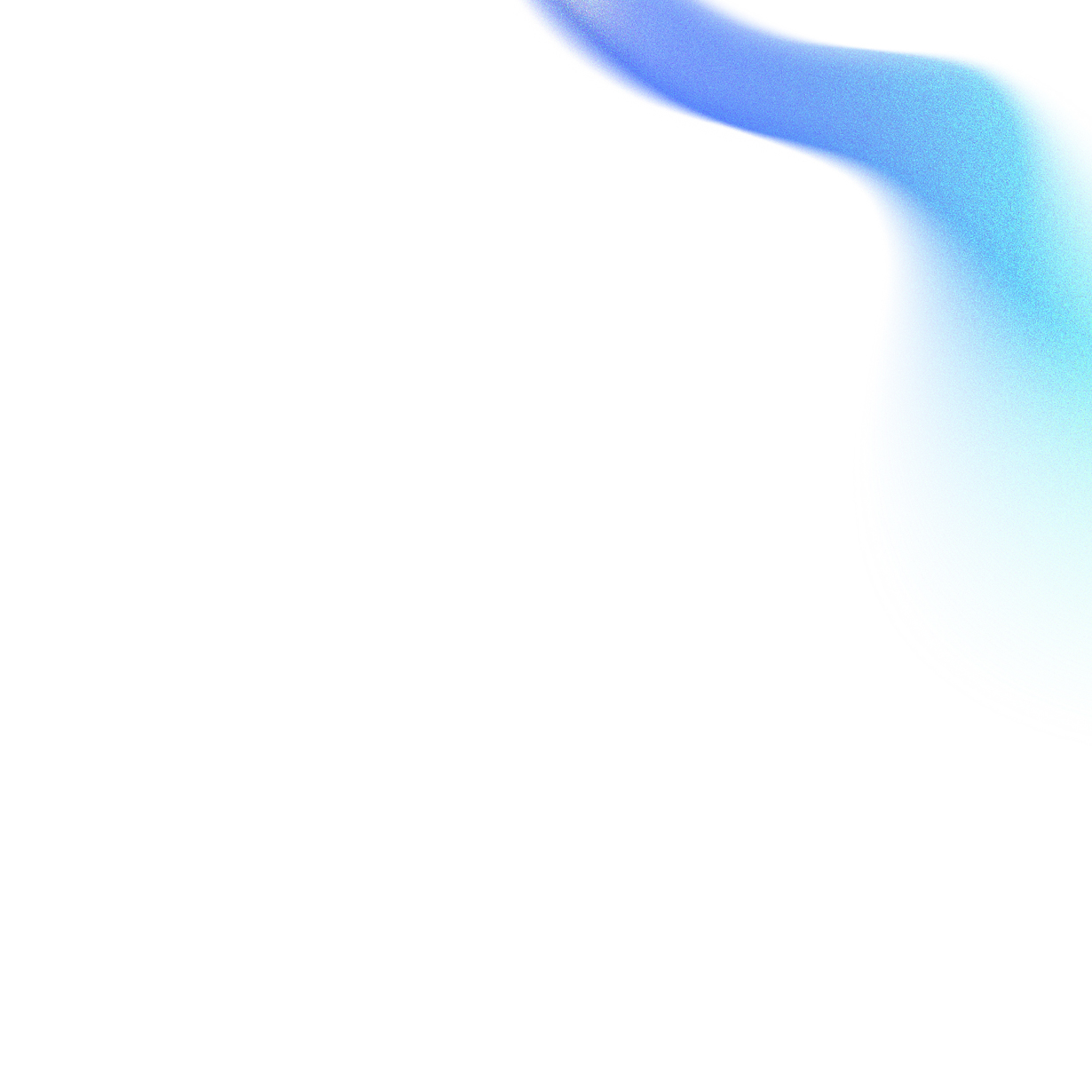
Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
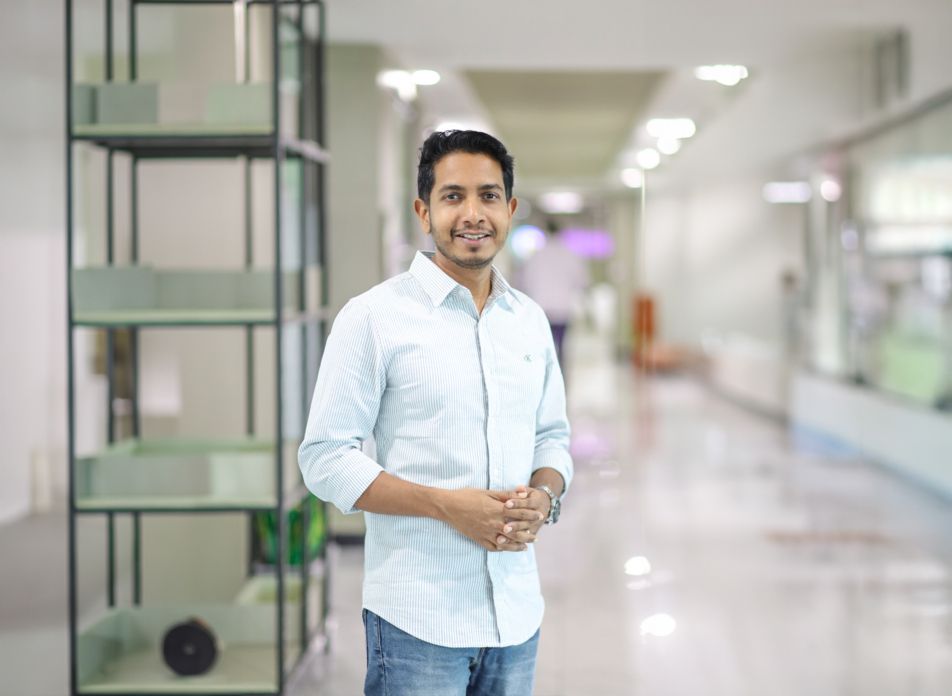