How to Handle Large Datasets in Databases with Laravel?
Dealing with large datasets in databases can be a challenge when building web applications. Loading millions of records can exhaust your system’s memory and slow down application performance. However, with the right Laravel development services, you can utilize several built-in tools that Laravel provides to efficiently manage and process large amounts of data without overloading your server.
This article will explore how to handle large database datasets while keeping your Laravel application responsive.
Issues with Large Database Datasets
When dealing with large datasets in a database, several key challenges arise:
- Memory Exhaustion: Loading all the records into memory at once can quickly hit system memory limits.
- Performance Bottlenecks: Querying and processing large datasets can significantly slow down the application.
- Time Efficiency: Iterating over a huge dataset in a single process is time-consuming and inefficient.
Laravel provides useful tools like chunking, cursor-based pagination, and batch processing to solve these issues.
Solutions for Handling Large Database Datasets in Laravel
1. Chunking Data with chunk()
One of the most effective ways to handle large datasets is by using Laravel’s chunk() method. This method allows you to process the data in smaller chunks, ensuring that only a portion of the records are loaded into memory at a time.
Basic Example of Chunking:
1 2 3 4 5 6 7 8 9 | DB::table('users')->chunk(1000, function ($users) { foreach ($users as $user) { // Process each user } }); |
Explanation:
- chunk(1000, function ($users)): This will fetch 1,000 rows from the users table at a time, process them, and then move to the next batch of 1,000 rows until all records are processed.
- The main benefit of using chunk() is that it prevents memory exhaustion by processing smaller sets of data.
2. Using Cursors with cursor()
While chunk() works well for many cases, it still loads a batch of data into memory. If you want to process data one record at a time with minimal memory usage, you can use Laravel’s cursor() method, which works similarly to PHP’s generator.
Basic Example of Using Cursor:
1 2 3 4 5 | foreach (DB::table('users')->cursor() as $user) { // Process each user } |
Explanation:
- cursor() allows you to iterate over a dataset one record at a time without loading all the records into memory. This method is especially useful when handling extremely large datasets.
- Each record is processed as it is retrieved, minimizing memory usage.
3. Pagination for User-Friendly Output
If you need to display large datasets in a user interface, using pagination ensures that data is loaded and displayed in chunks to prevent overwhelming users or exhausting server resources.
Basic Example of Pagination:
1 | $users = DB::table('users')->paginate(100); |
Explanation:
- paginate(100): This method will fetch 100 records per page, which can then be displayed in the application with pagination links. Laravel’s pagination system is efficient and provides built-in support for generating the pagination controls.
- This method is optimal when you need to display large datasets to users in a manageable way.
4. Batch Processing with Queues
For handling large datasets that need to be processed over time, such as sending emails to thousands of users or exporting data, batch processing with queues can help offload heavy operations and process them asynchronously in the background.
Basic Example of Using Queues for Batch Processing:
1 2 3 4 5 6 7 8 9 | use App\Jobs\ProcessUserData; $users = User::all(); foreach ($users as $user) { ProcessUserData::dispatch($user); } |
Explanation:
- This will queue each user for processing using Laravel’s queue system. You can then configure your queue workers to process the records in the background, avoiding blocking the main application and improving performance for end-users.
5. Optimizing Queries
Efficient handling of large datasets also depends on how you query the database. Optimizing queries can reduce load time and memory usage. A few key strategies include:
Eager Loading: Avoid the N+1 query problem by using with() to load related models.
1 | $users = User::with('posts')->get(); |
Indexing: Ensure your database tables are properly indexed for fast lookups.
1 | Schema::table('users', function (Blueprint $table) { $table->index('email'); }); |
Query Caching: Use caching techniques such as remember() to store frequently accessed results in memory.
1 | $users = DB::table('users')->remember(60)->get(); |
Advanced Techniques for Handling Large Datasets
1. Lazy Collections
Laravel’s Lazy Collections allows you to process large datasets while keeping memory usage low by only loading data into memory when necessary. This is useful for working with large files or streams of data.
Example of Lazy Collections:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | use Illuminate\Support\LazyCollection; LazyCollection::make(function () { $handle = fopen('large-file.csv', 'r'); while (($line = fgets($handle)) !== false) { yield str_getcsv($line); } })->each(function ($row) { // Process each row }); |
Benefit: Lazy collections only load one record at a time, keeping memory usage low.
2. Job Batching
If you’re processing large datasets using queues, you can leverage job batching to group multiple jobs into a single batch and process them simultaneously. Laravel provides built-in support for job batching.
Example of Job Batching:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | use App\Jobs\ProcessUserData; use Illuminate\Support\Facades\Bus; Bus::batch([ new ProcessUserData($user1), new ProcessUserData($user2), ])->then(function () { // All jobs completed })->dispatch(); |
Benefit: Job batching allows you to monitor the progress of batch jobs and handle successes or failures more efficiently.
Additional Tips
- Database Partitioning: For extremely large datasets, consider partitioning your tables to improve query performance and manageability.
- Horizontal Scaling: As your application grows, consider using database sharding or horizontal scaling to distribute your data across multiple databases.
Conclusion
Laravel offers several powerful tools to handle large datasets efficiently when dealing with database records. Whether you are fetching thousands of rows from the database, displaying large datasets to users, or processing data in the background, techniques like chunking, cursor-based retrieval, and queue-based batch processing ensure your application remains responsive and optimized.
If you need expert assistance in implementing these solutions, consider hiring a Laravel developer to help you manage large datasets effectively and ensure optimal performance.
Recent help desk articles
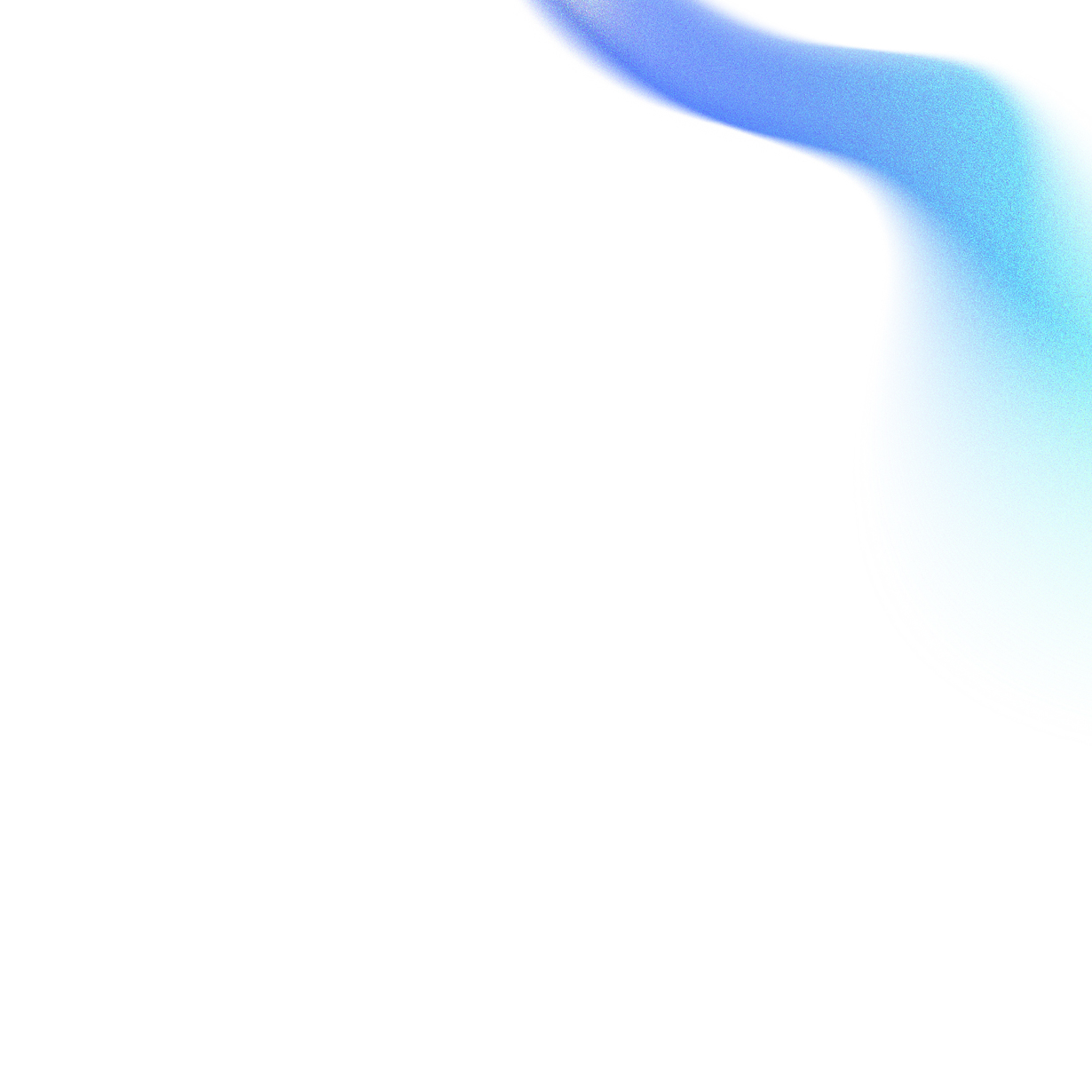
Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
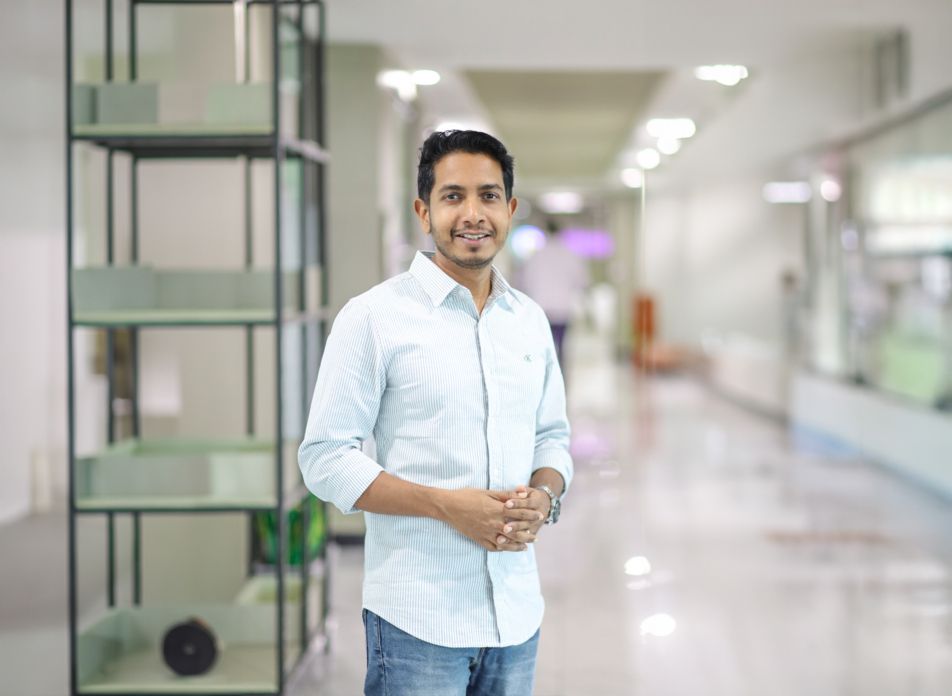