How do I programmatically invalidate the category listing cache in Shopware 6.5?
In Shopware 6.5, you may encounter situations where you need to programmatically invalidate the cache for category listings, especially when adding additional filters to product listings.
This guide will walk you through the process of resolving cache invalidation issues related to category listings.
Issue – Cache invalidation causing inaccuracy
When attempting to add a filter, such as a “category not in” filter, to the product listing using the ProductListingCriteriaEvent, you may notice that the persisted cache displays invalid results in the listing. This can occur for both logged-in and guest users, disrupting the accuracy of the product listing.
Solution – Programmatically invalidate category cache
To address this cache invalidation issue, you can implement a solution that programmatically invalidates the cache for category listings. Below is an example implementation using PHP and Symfony:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 | use ShopwareCoreFrameworkAdapterCacheCacheInvalidator; use ShopwareCoreContentProductSalesChannelListingCachedProductListingRoute; class ProductSubscriber implements EventSubscriberInterface { private $cacheInvalidator; public function __construct( CacheInvalidator $cacheInvalidator ) { $this->cacheInvalidator = $cacheInvalidator; } // Other event subscriber methods... ** Remove cache for specified category IDs. * @param array $categoryIds An array of category IDs to invalidate cache for. / public function removeCategoryCache($categoryIds) { // Build cache keys for each category ID $cacheKeys = array_map([CachedProductListingRoute::class, 'buildName'], $categoryIds); // Invalidate cache for each category $this->cacheInvalidator->invalidate($cacheKeys); } } |
In this solution
- We use the CacheInvalidator service provided by Shopware to invalidate cache entries.
- The removeCategoryCache method accepts an array of category IDs for which we want to invalidate the cache.
- We utilize CachedProductListingRoute::buildName method to construct cache keys for each category ID.
Finally, we invalidate the cache for each constructed cache key using the invalidate method of the CacheInvalidator service.
Conclusion
By implementing this solution, you ensure that the cache for category listings is properly invalidated when adding additional filters to product listings, thus resolving the issue of invalid results in the product listing. Looking for additional help? Contact us
Recent help desk articles
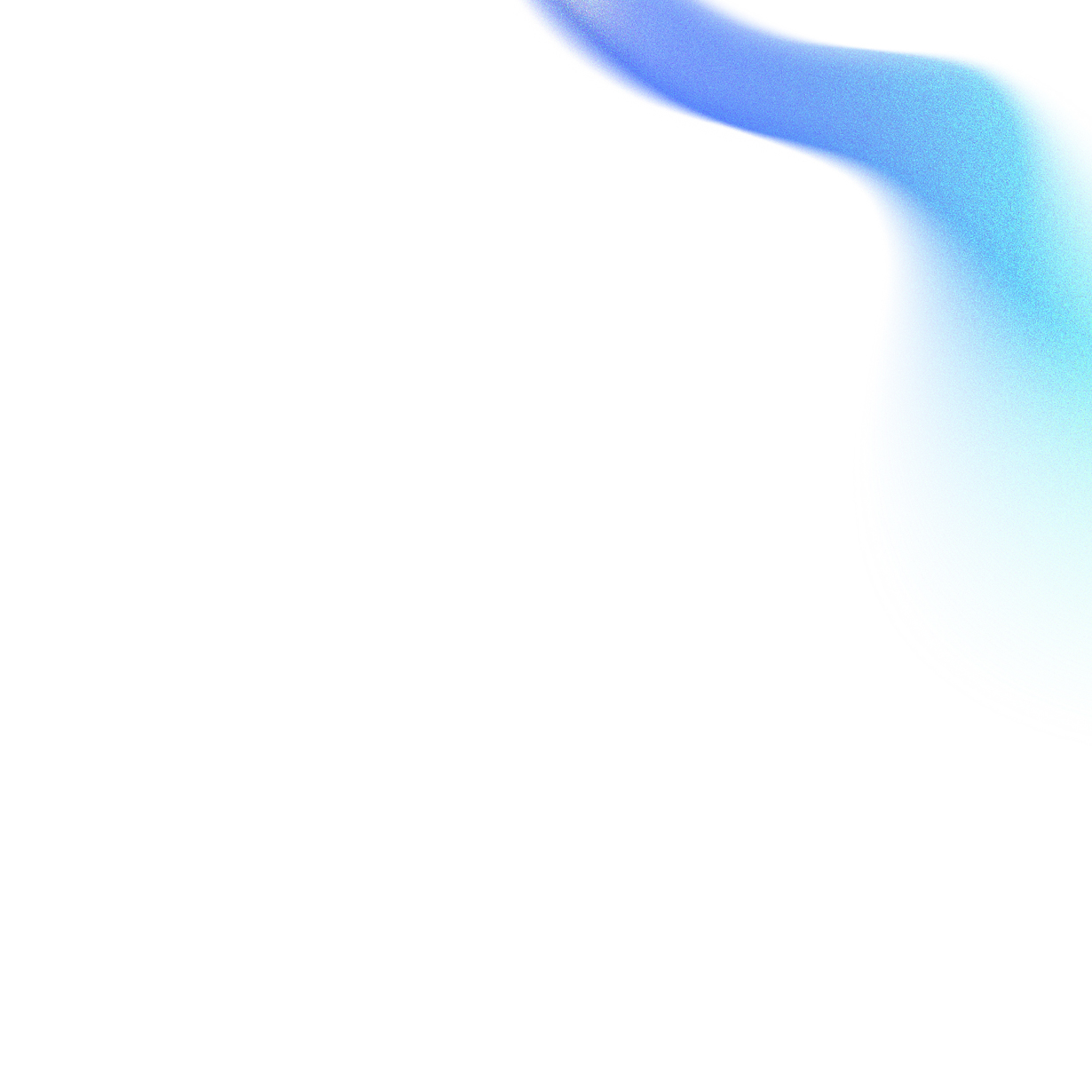
Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
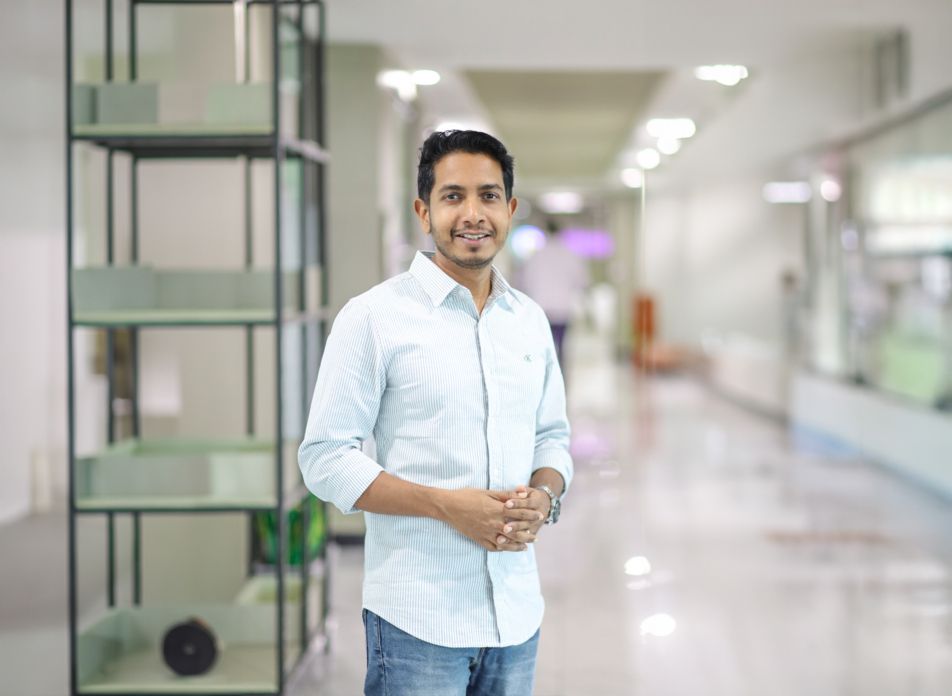