How to Upload Documents to an Order from a URL in Shopware 6?
As a developer working with Shopware 6, you may often need to integrate external systems seamlessly. One common task is uploading documents, such as invoices, from external URLs to Shopware orders using the platform’s API.
In this article, we will guide you through uploading an invoice (or other documents) to a Shopware 6 order from an external URL using an API. When you need to attach documents—like invoices—that were created or saved on other systems to Shopware orders, this method comes in handy.
Issue: Upload Invoice from Another Site via API
Sometimes, you may need to upload an invoice or document to a Shopware order from an external site. This can be accomplished using an API function to fetch the document from the URL and upload it to Shopware.
Steps to upload documents to order in Shopware 6
Here is the solution for uploading a document to an order in Shopware 6 using the following function: uploadDocuments($orderId, $fileUrl)
.
Step 1: Fetch the file from the external URL.
The function begins by fetching the file content from the external URL using PHP’s file_get_contents()
method.
1 2 3 | php $fileContent = file_get_contents($fileUrl); |
Step 2: Create a temporary file.
Once the content is fetched, the next step is to create a temporary file in which the fetched content will be stored.
1 2 3 4 5 6 7 | php $tempFile = tmpfile(); fwrite($tempFile, $fileContent); $tempFileMeta = stream_get_meta_data($tempFile); |
Step 3: Prepare the File Information Object.
Create a file object that includes important file details such as the path, original name, MIME type, and size. This information will be used to pass the file into Shopware’s media helper for upload.
1 2 3 4 5 6 7 8 9 10 11 | $file = new \stdClass(); $file->path = $tempFileMeta['uri']; $file->originalName = 'filename'; $file->mimeType = 'application/pdf'; $file->size = strlen($fileContent); $file->extension = pathinfo('filename', PATHINFO_EXTENSION); |
Step 4: Then upload the file to Shopware media.
Using the uploadMedia
function from Shopware’s media helper, the file is uploaded to the Shopware media system, returning a mediaId
that will be linked to the order document.
1 2 3 | php $mediaId = $this->mediaHelper->uploadMedia($file, $this->context); |
After the upload, close the temporary file:
1 2 3 | php fclose($tempFile); |
Step 5: Update order with uploaded document.
Now that the document is uploaded, you need to update the Shopware order with this document. This is done by passing the media ID and file type to the updateOrderDocuments()
function.
1 2 3 4 5 6 7 8 9 | $data = [ "mediaId" => $mediaId, "fileType" => $file->mimeType ]; $this->updateOrderDocuments($orderId, $data); |
Step 6: Create and associate document with order.
In the updateOrderDocuments()
function, the document repository is used to create a new document for the order. The document type is fetched based on the type (e.g., invoice) and the document is linked with the order.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | $documentData = [ 'documentTypeId' => $this->getDocumentTypeId('invoice'), 'fileType' => $data['fileType'] ?? 'application/pdf', 'orderId' => $orderId, 'config' => ['documentNumber' => (string)rand(1000, 9999)], 'deepLinkCode' => bin2hex(random_bytes(16)), 'documentMediaFileId' => $data['mediaId'], 'static' => false, 'sent' => false ]; $documentRepository->create([$documentData], $this->context); |
The function getDocumentTypeId()
fetches the document type based on the technical name provided (e.g., ‘invoice’):
1 | $documentTypeId = $this->getDocumentTypeId('invoice'); |
Full Code Solution
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 | public function uploadDocuments($orderId, $fileUrl) { $fileContent = file_get_contents($fileUrl); // Create a temporary file $tempFile = tmpfile(); fwrite($tempFile, $fileContent); $tempFileMeta = stream_get_meta_data($tempFile); // Prepare file details $file = new \stdClass(); $file->path = $tempFileMeta['uri']; $file->originalName = 'filename'; $file->mimeType = 'application/pdf'; $file->size = strlen($fileContent); $file->extension = pathinfo('filename', PATHINFO_EXTENSION); // Upload file to media $mediaId = $this->mediaHelper->uploadMedia($file, $this->context); // Close temp file fclose($tempFile); // Update order with document $data = [ "mediaId" => $mediaId, "fileType" => $file->mimeType ]; $this->updateOrderDocuments($orderId, $data); } private function updateOrderDocuments($orderId, $data) { $documentRepository = $this->container->get('document.repository'); $documentTypeId = $this->getDocumentTypeId('invoice'); $documentData = [ 'documentTypeId' => $documentTypeId, 'fileType' => $data['fileType'] ?? 'application/pdf', 'orderId' => $orderId, 'config' => ['documentNumber' => (string)rand(1000, 9999)], 'deepLinkCode' => bin2hex(random_bytes(16)), 'documentMediaFileId' => $data['mediaId'], 'static' => false, 'sent' => false ]; $documentRepository->create([$documentData], $this->context); } private function getDocumentTypeId($technicalName) { $documentTypeRepository = $this->container->get('document_type.repository'); $criteria = new Criteria(); $criteria->addFilter(new EqualsFilter('technicalName', $technicalName)); $documentTypeId = $documentTypeRepository->searchIds($criteria, $this->context)->firstId(); if (!$documentTypeId) { throw new \Exception("Document type '{$technicalName}' not found"); } return $documentTypeId; } ``` |
Conclusion
By following this guide, you can seamlessly upload and attach invoices or other documents from external URLs to orders in Shopware 6 via API integration. This allows for automated processing of documents from other systems, improving workflow efficiency. For expert services, contact a professional Shopware agency for smooth implementation.
Recent help desk articles
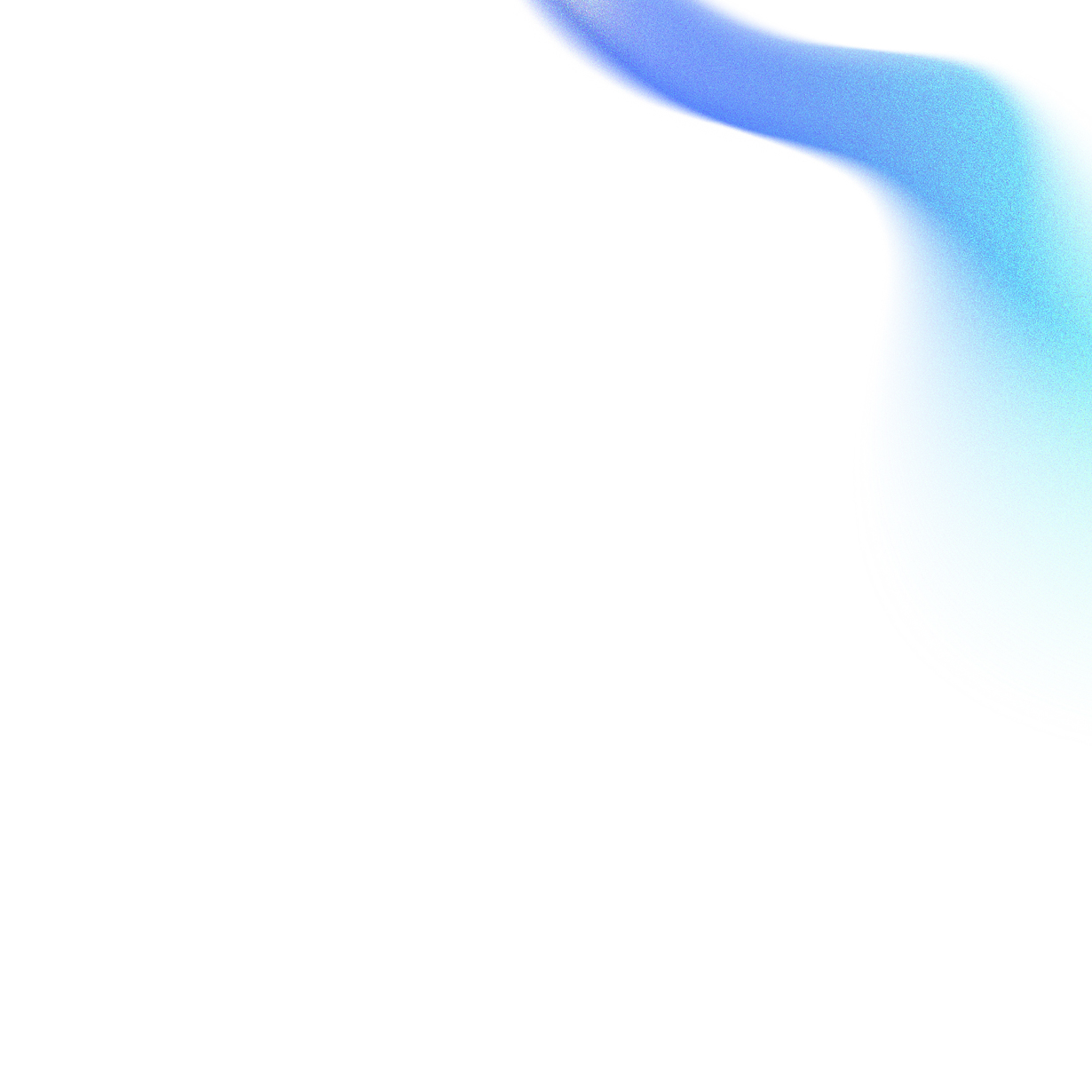
Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
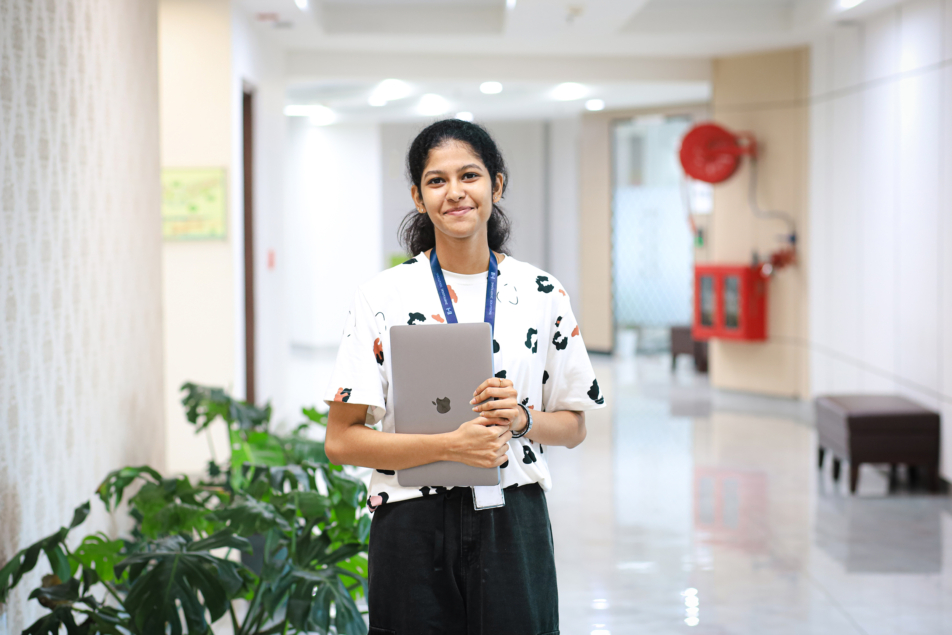